Till now we have seen different data types.
Variables of those data types are used to store the values.
Now assume that we want to store the marks of a group of students then instead of giving different variable name to the marks of different students we can give a common variable name to store the marks of this group of students.
Array can be used to do this.
An array is a group of related data items that share a common name.
Declaration of arrays:
Like any other variables, arrays must be declared before they are used.
The general form of array declaration is,
Data type variable name [size];
The data type specifies the type of element that will be contained in the array such as int, float or char and the size indicates the maximum number of elements that can be stored inside the array.
Variable name is the name given to the array.
For example:
int marks [100];
The above statement declares the marks to be an array containing 100 integer elements.
Each of the elements in the array is accessed with the help of an index or subscript.
In arrays the first element has the subscript as 0. So marks[0] represents the first element of the array marks, marks[1] represents second element, marks[2] represents third element and so on. Thus according to the above declaration the last subscript will be 99.
So any subscripts from 0 to 99 are valid.
One dimensional array: A list of items can be given one variable name using only one subscript and such a variable is called a single subscripted variable or a one dimensional array.
When we declare say int marks [4], the computer reserves 4 storage locations i.e., 8 bytes because marks is an integer array.
int marks [4];
We can store the values in each of the location as given below
marks [0] = 77;
marks [1] = 78;
marks [2] = 67;
marks [3] = 81;
The subscript of an array can be an integer constant, integer variables like i, j, k or expressions that yield integers.
Array elements are stored in contiguous memory locations.
Note: C performs no bounds checking and therefore care should be exercised to ensure that the array indices are within the declared limits. i.e. if we have declared an array of marks[50] then we should use only from marks[0] to marks[49] but we should not use any subscript which is above 49.
Similarly we can declare an array of real values as float array name[size]
Example:
float salary[100];
which declares salary as an array of 100 real elements.
Like wise we can declare an array of characters.
Example:
char strn [25] is an array named strn containing 25 character elements.
Note: When declaring character array we must allow an extra-element space for the null character ('\0').
Two-dimensional arrays:
These are used to represent table of values that is having more than one row and one column.
Syntax:
Data type variable name [row size] [column size];
Assume if roll number and marks of students as shown in the table below are to be stored then we can use an array say int marks[4][2];
Where 4 represents the number of rows and 2 represents the number of columns.
Col. 0 Col. 1
row 0 181 475
row 1 182 490
row 2 183 488
row 3 184 465
Because of the declaration as given above we can store 8 values.
We store roll number in the first column of the row and marks in the second column of the row.
Since memory does not contain rows and columns whether it is a one-dimensional or two-dimensional array the memory elements are stored in one contiguous location.
/*Program to read the roll numbers and marks of students and calculate the average marks obtained*/
#include
#include
void main ( )
{
int marks[4][2],sum,i,j;
float average;
sum = 0;
average = 0.0;
for (i=0; i<4;i++)
{
printf ("Enter the roll number & marks a student\n");
for (j=0; j<2; j++)
{
scanf ("%d",&marks[i][j]);
}
}
for (i=0; i<4; i++)
{
sum = sum + marks[i][1];
}
average = sum /4.0;
printf ("Average marks obtained by the students is %f\n ", average);
getch();
}
Output:
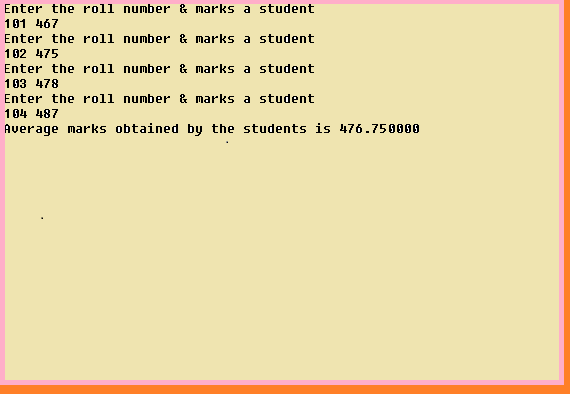
Initialization of arrays:
Array elements can be initialized in the same way as the ordinary variables when they are declared.
Syntax:
static data type array name[size] = {list of values}
Example:
static int marks[5] = {77, 87, 67, 53,}
The above statement will declare the variable marks as an array of size 5 and assign 77 to 0th element, 87 to 1st element, 67 to 2nd element, 53 to 3rd element, 47 to 4th element. If the number of values in the list is less than the number of elements then only that many elements will be initialized and the remaining elements will be set to zero automatically since the storage class is declared as static.
Example:
static float code[5] = {1.0, 2.0, 3.0};
The above statement will initialize first 3 elements to 1.0, 2.0 and 3.0 respectively and the rest 2 elements to zero .
When you are initializing the array elements the size may be omitted. In such cases a compiler allocates enough space for all initialized elements.
static int counter[ ] = {1, 1, 1};
The above statement declares an array named as counter for which compiler will allocate three locations each of two bytes because there are three elements inside the flower brackets. The initial value of each element is 1.
As long as we initialize every element in the array this approach works fine. Thus character arrays may be initialized as given below.
Example:
static char alphabets[ ] = {'x', 'y', 'z'};
Initializing 2 dimensional arrays:
Similar to one dimensional array, two dimensional arrays may be initialized by following their declaration with a list of initial value enclosed in braces.
Example:
The examples given below shows the different types of initialization of two-dimensional arrays.
static int table[2 ][2 ] = {1, 2, 3, 4};
In the case shown above first two elements represent two columns of first row and the next two elements represents two columns of next row.
static int table[ 2 ][ 2 ] ={{1, 2}, {3, 4}};
In the above case two elements within the brace represent two columns of first row and the next two elements within the brace represents two columns of next row. The same concept can be extended to the next example.
static int table[ 2 ][ 2 ] ={
{1, 2},
{3, 4}
};
In the following example the first two columns of first row will be initialized to 1 and 2. The first column of the second row will be initialized to 3 and the second column will be initialized to 0.
static int table [ 2 ] [ 2 ] ={
{1, 2}
{3}
};
/* Program to perform addition and subtraction on matrices*/
#include
<stdio.h>
#include<conio.h>
void main( )
{
int a[10][10], b[10][10], c[10][10],i, j,m,n,p,q,choice;
char ch;
clrscr();
printf("Enter the order of the matrix a \n");
scanf("%d%d",&m,&n);
printf("Enter the order of the matrix b \n");
scanf("%d%d",&p,&q);
if((m!=p)||(n !=q))
{
printf("Order of matrix are not same so addition or subtraction is not possible \n");
}
else
{
printf("Enter the elements of the matrix a \n");
for(i=0; i<m;i++)
{
for(j=0; j<n;j++)
{
scanf("%d",&a[i][j]);
}
}
printf("Enter the elements of the matrix b \n");
for(i=0; i<p;i++)
{
for(j=0; j<q;j++)
{
scanf("%d", &b[i][j]);
}
}
do{
printf("\t 1. Addition of matrix\n");
printf("\t 2. Subtraction of matrix\n");
printf(" Press 1 for Addtion and 2 for Subtraction---> ");
scanf("%d",&choice);
switch(choice)
{
case 1:
for(i=0; i<m;i++)
for(j=0; j<n;j++)
{
c[i][j]= a[i][j] + b[i][j];
}
}
printf("The resultant matrix of addition is\n");
break;
case 2:
for(i=0; i<m;i++)
{
for(j=0; j<n;j++)
{
c[i][j]= a[i][j] - b[i][j];
}
}
printf("The resultant matrix of subtraction is\n");
break;
}
for(i=0; i<m;i++)
{
for(j=0; j<n;j++)
{
printf("%d\t", c[i][j]);
}
printf("\n");
}
flushall();
printf("Press 'N' to terminate or any key to continue---> ");
ch=getchar();
} while(ch !='N');
}
getch();
}
Output 1:
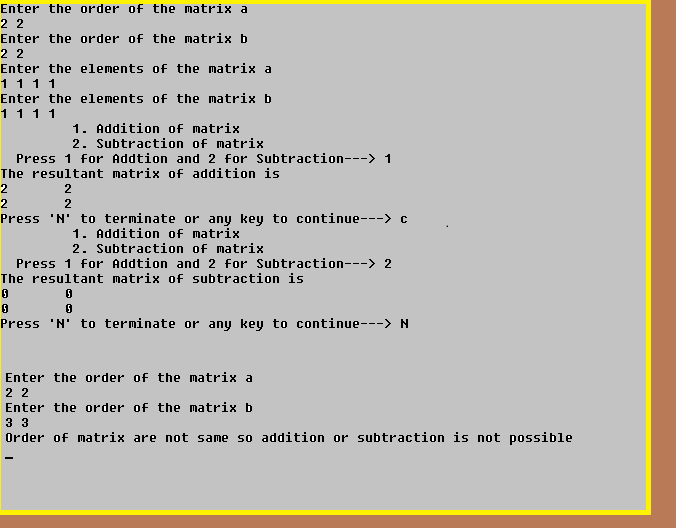
Output 2:
/*C Program to multiply 2 matrices a & b and to store the result in c and to find the transpose and norm of c*/
#include
#include
#include
void main( )
{
int a[10][10], b[10][10], c[10][10], d[10][10],i, j, k, p, q, m, n, sum;
float norm;
clrscr( );
printf("Enter the order of the matrix a \n");
scanf("%d%d", &m,&n);
printf("Enter the order of the matrix b \n");
scanf("%d%d", &p,&q);
if(m!=p)
{
printf("Matrix multiplication is not possible \n");
}
else
{
printf("Enter the elements of the matrix a \n");
for(i=0; i
{
for(j=0; j
{
scanf("%d",&a[i][j]);
}
}
printf("Enter the elements of the matrix b \n");
for(i=0; i
{
for(j=0; j
{
scanf("%d",&b[i][j]);
}
}
for(i=0; i
{
for(j=0; j
{
c[i][j]=0;
for(k=0; k
{
c[i][j]= c[i][j]+ a[i][k]* b[k][j];
}
}
}
printf("The resultant matrix \n");
for(i=0; i
{
for(j=0; j
{
printf("%d\t", c[i][j]);
}
printf("\n");
}
for(i=0; i
{
for(j=0; j
{
d[i][j]= c[j][i];
}
}
printf("The transpose of the matrix c is \n");
for(i=0; i
{
for(j=0; j
{
printf("%d\t",d[i][j]);
}
printf("\n");
}
sum=0;
for(i=0; i
{
for(j=0; j
{
sum=sum+ c[i][j]* c[i][j];
}
}
norm=sqrt(sum);
printf("The norm of the given matrix is %f \n",norm);
}
getch();
}
Output
/*Program to count the number of words in a string */
#include
<stdio.h>
#include
<conio.h>
void main ()
{
char strn [100];
int word, i;
i = 0; word = 0;
printf ("Enter any string\n");
scanf("%[^\n]",strn);
while (strn[i]!= '\0')
{
if ((strn[i] == ' ' && strn[i+1]!= ' ') || strn[i+1] == '\0')
{
word++;
}
i++;
}
printf ("Number of words present in the entered string is %d\n", word);
getch();
}
Output 1
C Decision Making & Looping<< Previous
Next >> Handling of Character Strings
Our aim is to provide information to the knowledge
seekers.
Support us generously