C functions can be classified into two categories namely library functions and user defined functions. The main distinction between these two categories is that library functions are not required to be written by us whereas a user defined function has to be developed by the user at the time of writing a program.
Whenever we develop our program we write main() function. This is a user defined function. Every program must have a main function to indicate where the program has to begin its execution.
A function is a self-contained block of code that performs a particular task. Once a function has been designed and packed, it can be treated as a black box that takes some data from the main program and returns a value. The inner details of operation are invisible to the rest of the program.
The general form of the function is:
return type function name (argument list)
argument declaration;
{
local variable declaration;
executable statements;
:
:
return (expression);
}
Argument list and argument declaration are optional, it depends on the calling function. Local variable declaration is required only when any local variable are used in the function. A function may or may not send back any value to the calling function. If it does, it does it through the return statement. While it is possible to pass to the called function, any number of values, the called function can return one value per call at the most. The return statement can take one of the following forms
return;
or
return (expression);
The first form does not return value. It transfers the control back to the calling function. The second one returns the value of the expression to the calling function.
The return statement is the mechanism for returning a value to the calling function.
This is also an optional statement and its absence indicates that no value is being returned to the calling function.
Note: If a function is not returning any value to the calling function then it should be specified in the return type.
We have to specify the return type as void.
By default the return type of a function is int.
So if a function is returning a value and it is not an integer then it should be specified in the function header and it should specify the proper data type.
This information is also required to be specified in the beginning of the program.
Category of user defined functions:
Depending on the conditions like whether the arguments are passed to the called function and whether the called function is returning the value back to the calling function, the functions are categorized into following categories.
(i) Functions without arguments and without any return value.
In this the calling function is not passing any arguments to the called function and the called function is not returning back any value to the calling function.
Example:
#include<stdio.h>
void main ( )
{
void message();
printf ("I am in MGM College\n");
message ( );
}
void message ( )
{
printf ("I am in C class \n');
}
Output:
In the above program the function message() is called from main(). The function main() is not passing any value to the function message() and function message() is also not returning back any value to the function main().
(ii) Functions with arguments and without any return value.
In this the calling function is passing one or more arguments to the called function and the called function is not returning back any values to the calling function.
#include<stdio.h>
void main ()
{
int a, b;
void add();
a = 5;
b = 6;
add(a,b);
}
void add (x,y)
int x,y;
{
int z;
z = x+y;
printf ("Result is %d \n", z);
}
In the above program the function main() passes two arguments a and b to the function add(). But the function add() does not return any value back to the function main().
Note :In the above example add() is called function and main() is the calling function.
(iii) Functions with arguments and with return value.
In this the calling function is passing one or more arguments to the called function and the called function will return back one value to the calling function.
# include
<stdio.h>
void main ( )
{
int a, b, c;
int add();
a = 5;
b = 6;
c = add (a, b);
printf ("In main %d\n",c);
}
add (x, y)
int x, y;
{
int z;
z = x+y;
printf ("Result in function %d\n",z);
return (z);
}
In the above program the function main() passes two arguments a and b to function add() and the values are received in the function add() through the variables x and y. The variable z is local to the function add(). The function add() returns the result back to the main() through the variable z and it is received at the function main() though the variable c.
Output
Note 1: In the above program, the arguments a and b are known as actual arguments and arguments x and y are known as formal arguments. The values of actual arguments are copied to formal arguments when the functions is called.
Note2:Wherever function is not returning any value back to the main function we have used the return type as void. This aspect is explained in the next section.
Overriding the default return type:
By default C function returns a value of the type int when no other type is specified explicitly. When no other type is specified then it is assumed that the function is going to return a value of type int to the calling function.
We must do two things to enable calling function to receive a non-integer
value from a called function.
(1) The explicit type specifier corresponding to the data type required must be mentioned in the function header.
The Syntax is:
return type specifier function name (argument list)
declaration of argument list;
{
local variable declaration;
executable statements;
}
The return type specifier tells the compiler, the type of the data the function is to return.
(2) The called function must be declared at the start of the body in the calling function like any other variable. This is to tell the calling function, the type of data that the function is actually returning.
Example1:
# include
<stdio.h>
# include
<conio.h>
void main ( )
{
float a, radius;
float area( );
printf(“Enter the radius\n”);
scanf("%f", &radius);
a = area (radius);
printf ("Area of circle is : %f\n",a);
getch ( );
}
Function:
float area (r)
float r;
{
float ar;
ar = 3.14 *r * r;
return (ar);
}
Output:
In the above program the function area() which calculates the area of the circle and returns the value of type float to the calling function main(). So the return type specifier is mentioned in the function header as float it is given by the statement float area(r);. It is also specified in the calling function main() that the type of the value the function area() is going to return is float with statement float area(); after the declaration of the variables.
Example 2:
void main ( )
{
void message( );
printf ("I am in MGM College\n");
message ( );
}
void message ( )
{
printf ("I am in C class\n");
printf ("I am enjoying C programming language\n");
printf("I am dreaming about writing good programs in C\n");
}
Output:
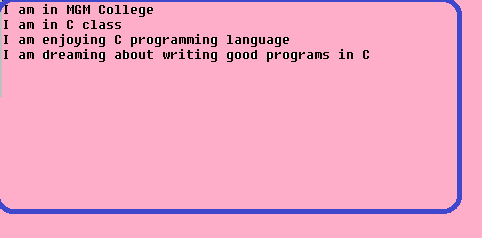
Similarly in the above program the function message() which just display the messages does not return any value to the calling function main(). So the return type specifier is mentioned in the function header as void as it is given by the statement void message();. It is also specified in the calling function main() that the function message() is not going to return any value with the statement void message(); in the beginning of the function main.
Note:
Void is the keyword which is used when a function is not returning any value to the calling function.
Scope Rule of functions:
Consider the following example:
# include
<stdio.h>
void main ( )
{
int i;
void display();
i= 80;
display(i);
}
void display (j)
int j;
{
int k;
k = 25;
printf("j = %d\n", j);
printf("k = %d\n", k);
}
Output
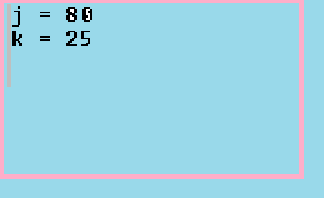
In the program it is necessary to pass the value of the variable i to the function display() and it is not automatically available to the function display. Because by default, the scope of the variable is local to the function in which it is defined. The presence of variable i is known only to the function main() and not to any other function
.
Similarly, the variable k is local to the function display() and hence it is not available to the function main(). That is why to make the value of i available to display(), we have to explicitly pass it to the function display. Likewise, if we want k to be available to the function main(), we will have to return it to main(), using the return statement.
So In general, we can say that the scope of a variable is local to the function in which it is defined.
/*C Program to print the GCD of 4 given numbers using function to calculate the GCD of two numbers*/
#include <stdio.h>
#include
<conio.h>
void main( )
{
int a, b, c, d, a1, a2, a3;
int gcd();
clrscr( );
printf("Enter the number \n");
scanf ("%d %d %d %d", &a, &b, &c, &d);
a1=gcd(a,b);
a2=gcd(c,d);
a3=gcd(a1, a2);
printf("GCD of 4 numbers is %d \n",a3);
getch( );
}
int gcd (p,q)
int p, q;
{
int g;
while (p != q)
{
if (p>q)
{
p=p - q;
g = p;
}
else
{
q = q - p;
g = q;
}
}
if ( p == q)
g = p;
return (g);
}
Output
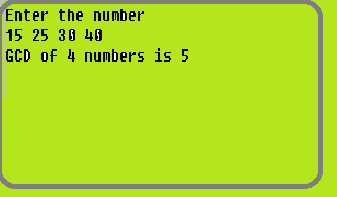
/* C program to perform computation on matrices
1. addition
2. subtraction
3. multiplication*/
#include
<stdio.h>
#include<conio.h>
void main( )
{
int a[10][10], b[10][10], c[10][10],i, j, m, n, p,q, choice, flg=0, ch;
void sum();
void sub();
void mul();
clrscr();
printf ("Enter the order of the first matrix\n");
scanf ("%d%d", &m,&n);
flushall( );
printf ("Enter the order of the second matrix\n");
scanf ("%d%d", &p,&q);
flushall( );
printf ("Enter the elements of the first matrix\n");
for (i=0; i<m;i++)
{
for (j=0; j<n;j++)
{
scanf("%d", &a[i][j]);
}
}
printf ("Enter the elements of the second matrix\n");
for (i=0; i<p;i++)
{
for (j=0; j<q;j++)
{
scanf("%d", &b[i][j]);
}
}
do
{
printf("1)Addition \t");
printf("2)Subtraction \t");
printf("3)Multiplication ");
printf("Enter Your Choice-->");
scanf("%d", &choice);
switch(choice)
{sum
case 1:
printf("Matrix Addition and ");
if((m == p) && (n == q))
{
(a, b, c, m, n);
flg = 1;
}
else
printf("Order of matrix do not match so addition is not possible \n");
break;
case 2:
printf("Matrix Subtraction and ");
if((m == p) && (n == q))
{
sub(a, b, c, m, n);
flg = 1;
}
else
printf("Order of matrix do not match so matrix subtraction is not possible \n");
break;
case 3:
printf("Matrix Multiplication and ");
if((n== p))
{
mul(a, b, c, m, n,q);
flg = 1;
}
else
printf("Order of matrix do not match so matrix multiplication is not possible \n");
break;
default: printf("Wrong Choice");
}
if (flg == 1)
{
printf("The resultant matrix is \n");
for (i=0; i<;i++)
{
for (j=0; j<q;j++)
{
printf("%d\t",c[i][j]);
}
printf("\n");
}
}
printf("Press 'Y' to continue and any other key to terminate --->");
flushall();
ch=getchar();
}
while (ch == 'Y');
getch( );
}
/* Function for matrix addition */
void sum(d, e, h, f, g)
int d[10][10], e[10][10], h[10][10], f, g;
{
int i, j;
for (i=0; i<f;i++)
{
for (j=0; j<g;j++)
{
h[i][j] = d[i][j] + e[i][j];
}
}
}
/* Function for matrix subtraction */
void sub(d, e, h, f, g)
int d[10][10], e[10][10], h[10][10], f, g;
{
int i, j;
for (i=0; i<f;i++)
{
for (j=0; j<g;j++)
{
h[i][j] = d[i][j] - e[i][j];
}
}
}
/* Function for matrix multiplication */
void mul(d, e, h, f, g,l)
int d[10][10], e[10][10], h[10][10], f, g,l;
{
int i, j, k;
for (i=0; i<f;i++)
{
for (j=0; j<1;j++)
{
h[i][j] = 0;
for (k=0; k<g;k++)
{
h[i][j] = h[i][j] + d[i][k] * e[k][j];
}
}
}
}
Output
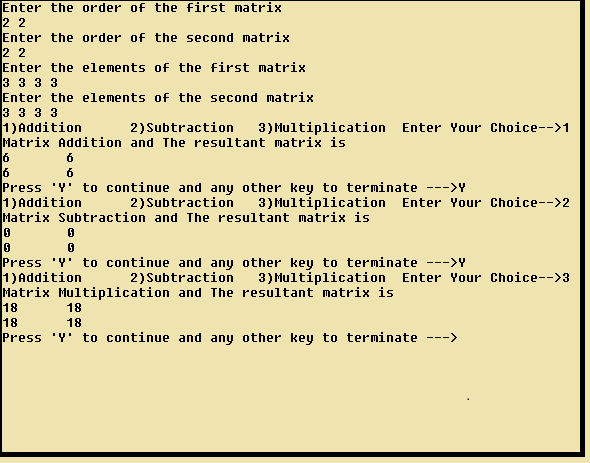
Recursion:
It is a process by which a function calls repeatedly the same function until some specified condition has been satisfied.
The process is used for repetitive computation in which each action is started in terms of previous result.
Example:
Program to find the factorial of an Integer using recursion
#include
<stdio.h>
#include
<conio.h>
void main()
{
int n;
long int fct;
long int factorial(int);
printf("Enter the integer\n");
scanf("%d",&n);
fct=factorial(n);
printf("Factorial of %d is %ld\n", n,fct);
getch();
}
long int factorial(m)
int m;
{
long int f;
if(m==0)
return(1);
else
}
f= m * factorial(m-1);
return(f);
Output:
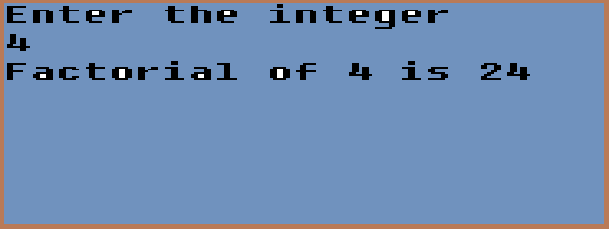
Note:
There should be a terminating condition in the recursive function to come out of the function.
If recursive function contains local variables a different set of local variables will be created during each call.
The names of the local variables of course will always be same as declared within the function. However the variable will represent set of values each time a function is executed. Each set of values will be stored on the stack so that they can be popped off the stack and used.
Program to generate fibanacci series using a recursive function
#include
<stdio.h>
#include
<conio.h>
void main()
{
int i,n,fib;
int fibanacci(int);
printf("Enter the number of terms for series\n");
scanf("%d",&n);
printf("Fibanacci series with %d terms\n",n);
for(i=1; i<=n; i++)
{
fib=fibanacci(i);
printf("%d\n", fib);
}
getch();
}
fibanacci( m)
int m;
{
int f;
if(m==1)
return(0);
if( m==2)
return(1);
f=fibanacci(m-1) + fibanacci( m-2);
return(f);
}
Output:
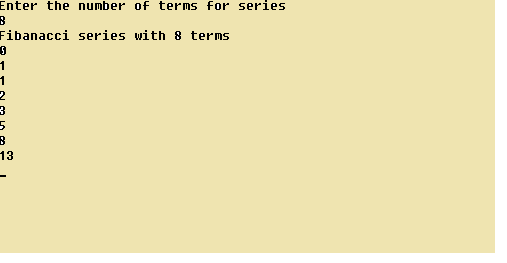
Searching And Sorting << Previous
Next >>Files
Our aim is to provide information to the knowledge
seekers.
Support us generously